آموزش طرح بندی خودکار در برنامه نویسی iOS
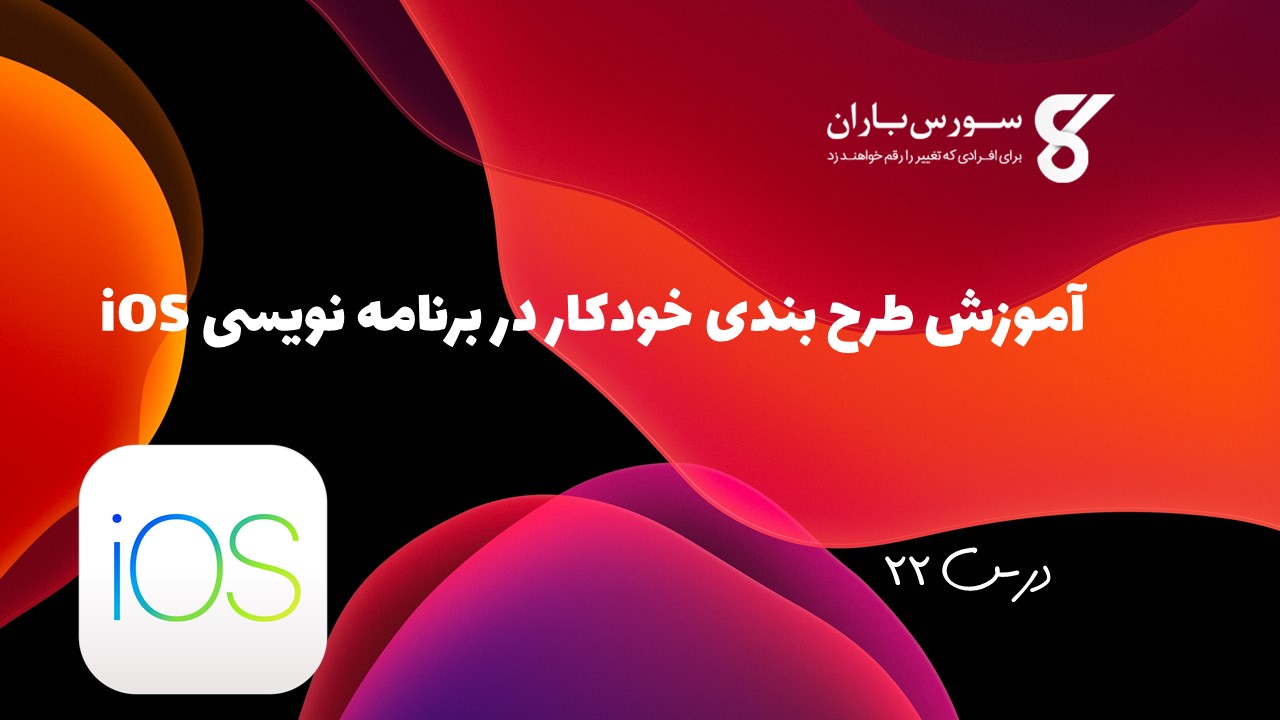
آموزش طرح بندی خودکار در برنامه نویسی iOS
در این درس از مجموعه آموزش برنامه نویسی سایت سورس باران، به آموزش طرح بندی خودکار در برنامه نویسی iOS خواهیم پرداخت.
طرح بندی خودکار در iOS 6.0 معرفی شد. وقتی از طرح بندی خودکار استفاده می کنیم ، هدف استقرار ما باید 6.0 و بالاتر باشد. طرح بندی خودکار به ما کمک می کند رابط هایی ایجاد کنیم که می توانند برای چندین جهت و چندین دستگاه استفاده شوند.
هدف از مثال
ما دو دکمه را اضافه خواهیم کرد که در فاصله مشخصی از مرکز صفحه قرار می گیرند. همچنین سعی خواهیم کرد یک قسمت متن قابل تغییر مجدد اضافه کنیم که از فاصله مشخصی از بالای دکمه ها قرار داده شود.
رویکرد
ما یک قسمت متن و دو دکمه به همراه محدودیت های آنها در کد اضافه خواهیم کرد. محدودیت های هر عنصر UI ایجاد و به نمای فوق العاده اضافه می شود. برای اینکه نتیجه دلخواه را بدست آوریم، باید تغییر اندازه خودکار را برای هر یک از عناصر رابط کاربری که اضافه می کنیم غیرفعال کنیم.
مراحل طرح بندی خودکار در برنامه نویسی iOS
مرحله 1 – یک برنامه ساده مبتنی بر نمایش ایجاد کنید.
مرحله 2 – ما فقط ViewController.m را ویرایش خواهیم کرد و به شرح زیر است –
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 |
#import "ViewController.h" @interface ViewController () @property (nonatomic, strong) UIButton *leftButton; @property (nonatomic, strong) UIButton *rightButton; @property (nonatomic, strong) UITextField *textfield; @end @implementation ViewController - (void)viewDidLoad { [super viewDidLoad]; UIView *superview = self.view; /*1. Create leftButton and add to our view*/ self.leftButton = [UIButton buttonWithType:UIButtonTypeRoundedRect]; self.leftButton.translatesAutoresizingMaskIntoConstraints = NO; [self.leftButton setTitle:@"LeftButton" forState:UIControlStateNormal]; [self.view addSubview:self.leftButton]; /* 2. Constraint to position LeftButton's X*/ NSLayoutConstraint *leftButtonXConstraint = [NSLayoutConstraint constraintWithItem:self.leftButton attribute:NSLayoutAttributeCenterX relatedBy:NSLayoutRelationGreaterThanOrEqual toItem:superview attribute: NSLayoutAttributeCenterX multiplier:1.0 constant:-60.0f]; /* 3. Constraint to position LeftButton's Y*/ NSLayoutConstraint *leftButtonYConstraint = [NSLayoutConstraint constraintWithItem:self.leftButton attribute:NSLayoutAttributeCenterY relatedBy:NSLayoutRelationEqual toItem:superview attribute: NSLayoutAttributeCenterY multiplier:1.0f constant:0.0f]; /* 4. Add the constraints to button's superview*/ [superview addConstraints:@[ leftButtonXConstraint, leftButtonYConstraint]]; /*5. Create rightButton and add to our view*/ self.rightButton = [UIButton buttonWithType:UIButtonTypeRoundedRect]; self.rightButton.translatesAutoresizingMaskIntoConstraints = NO; [self.rightButton setTitle:@"RightButton" forState:UIControlStateNormal]; [self.view addSubview:self.rightButton]; /*6. Constraint to position RightButton's X*/ NSLayoutConstraint *rightButtonXConstraint = [NSLayoutConstraint constraintWithItem:self.rightButton attribute:NSLayoutAttributeCenterX relatedBy:NSLayoutRelationGreaterThanOrEqual toItem:superview attribute: NSLayoutAttributeCenterX multiplier:1.0 constant:60.0f]; /*7. Constraint to position RightButton's Y*/ rightButtonXConstraint.priority = UILayoutPriorityDefaultHigh; NSLayoutConstraint *centerYMyConstraint = [NSLayoutConstraint constraintWithItem:self.rightButton attribute:NSLayoutAttributeCenterY relatedBy:NSLayoutRelationGreaterThanOrEqual toItem:superview attribute: NSLayoutAttributeCenterY multiplier:1.0f constant:0.0f]; [superview addConstraints:@[centerYMyConstraint, rightButtonXConstraint]]; //8. Add Text field self.textfield = [[UITextField alloc]initWithFrame: CGRectMake(0, 100, 100, 30)]; self.textfield.borderStyle = UITextBorderStyleRoundedRect; self.textfield.translatesAutoresizingMaskIntoConstraints = NO; [self.view addSubview:self.textfield]; //9. Text field Constraints NSLayoutConstraint *textFieldTopConstraint = [NSLayoutConstraint constraintWithItem:self.textfield attribute:NSLayoutAttributeTop relatedBy:NSLayoutRelationGreaterThanOrEqual toItem:superview attribute:NSLayoutAttributeTop multiplier:1.0 constant:60.0f]; NSLayoutConstraint *textFieldBottomConstraint = [NSLayoutConstraint constraintWithItem:self.textfield attribute:NSLayoutAttributeTop relatedBy:NSLayoutRelationGreaterThanOrEqual toItem:self.rightButton attribute:NSLayoutAttributeTop multiplier:0.8 constant:-60.0f]; NSLayoutConstraint *textFieldLeftConstraint = [NSLayoutConstraint constraintWithItem:self.textfield attribute:NSLayoutAttributeLeft relatedBy:NSLayoutRelationEqual toItem:superview attribute: NSLayoutAttributeLeft multiplier:1.0 constant:30.0f]; NSLayoutConstraint *textFieldRightConstraint = [NSLayoutConstraint constraintWithItem:self.textfield attribute:NSLayoutAttributeRight relatedBy:NSLayoutRelationEqual toItem:superview attribute: NSLayoutAttributeRight multiplier:1.0 constant:-30.0f]; [superview addConstraints:@[textFieldBottomConstraint , textFieldLeftConstraint, textFieldRightConstraint, textFieldTopConstraint]]; } - (void)didReceiveMemoryWarning { [super didReceiveMemoryWarning]; // Dispose of any resources that can be recreated. } @end |
نکاتی که باید توجه داشته باشید
در مراحل علامت گذاری شده 1 ، 5 و 8 ، ما به ترتیب از طریق برنامه ریزی به ترتیب دو دکمه و یک قسمت متن اضافه کردیم.
در بقیه مراحل، محدودیت هایی ایجاد کردیم و آن محدودیت ها را به super view های مربوطه اضافه کردیم ، که در واقع self-view هستند. محدودیت های یکی از دکمه های سمت چپ به شرح زیر است –
1 2 3 4 |
NSLayoutConstraint *leftButtonXConstraint = [NSLayoutConstraint constraintWithItem:self.leftButton attribute:NSLayoutAttributeCenterX relatedBy:NSLayoutRelationGreaterThanOrEqual toItem:superview attribute: NSLayoutAttributeCenterX multiplier:1.0 constant:-60.0f]; |
ما constraintWithItem و toItem داریم که تصمیم می گیرند بین کدام عناصر UI محدودیت ایجاد کنیم. ویژگی تعیین می کند که این دو عنصر بر چه اساسی به هم پیوند خورده اند. “relatedBy” تصمیم می گیرد که ویژگی ها چه تاثیری بین عناصر داشته باشند. ضریب ضریب ضرب است و ثابت به ضرب اضافه می شود.
در مثال بالا، X دکمه سمت چپ با توجه به مرکز نمای فوقانی همیشه بزرگتر یا برابر با -60 پیکسل است. به همین ترتیب ، محدودیت های دیگر نیز تعریف شده است.
لیست جلسات قبل آموزش برنامه نویسی iOS
- آموزش برنامه نویسی iOS
- شروع آموزش برنامه نویسی iOS
- آموزش تنظیمات محیطی iOS
- آموزش objective C در بزنامه نویسی iOS
- آموزش ایجاد برنامه آیفون در برنامه نویسی iOS
- آموزش اکشن و خروجی در برنامه نویسی iOS
- آموزش Delegate در iOS
- آموزش عناصر UI در برنامه نویسی iOS
- آموزش ایجاد شتاب سنج در برنامه نویسی iOS
- برنامه های جهانی در برنامه نویسی iOS
- آموزش مدیریت دوربین در برنامه نویسی iOS
- آموزش مدیریت مکان در برنامه نویسی iOS
- آموزش پایگاه داده SQLite در برنامه نویسی iOS
- آموزش ارسال ایمیل در برنامه نویسی iOS
- آموزش صدا و تصویر در برنامه نویسی iOS
- آموزش مدیریت فایل در برنامه نویسی iOS
- آموزش دسترسی به نقشه ها در برنامه نویسی iOS
- آموزش خرید درون برنامه ای در برنامه نویسی iOS
- آموزش ادغام iAd در برنامه نویسی iOS
- آموزش GameKit در برنامه نویسی iOS
- آموزش استوری بورد در برنامه نویسی iOS
دیدگاه شما